從 flutter_driver 遷移
本頁說明如何將現有使用 flutter_driver
的專案遷移到 integration_test
套件,以執行整合測試。
使用 integration_test
的測試與小工具測試中使用的方法相同。
如需 integration_test
套件的介紹,請查看整合測試指南。
入門範例專案
#本指南中的專案是一個小型範例桌面應用程式,具有以下功能:
- 在左側,有一個植物列表,使用者可以捲動、點擊和選擇。
- 在右側,有一個詳細資訊畫面,顯示植物名稱和物種。
- 在應用程式啟動時,如果未選擇任何植物,則會顯示要求使用者選擇植物的文字。
- 植物列表是從位於
/assets
資料夾中的本機 JSON 檔案載入的。
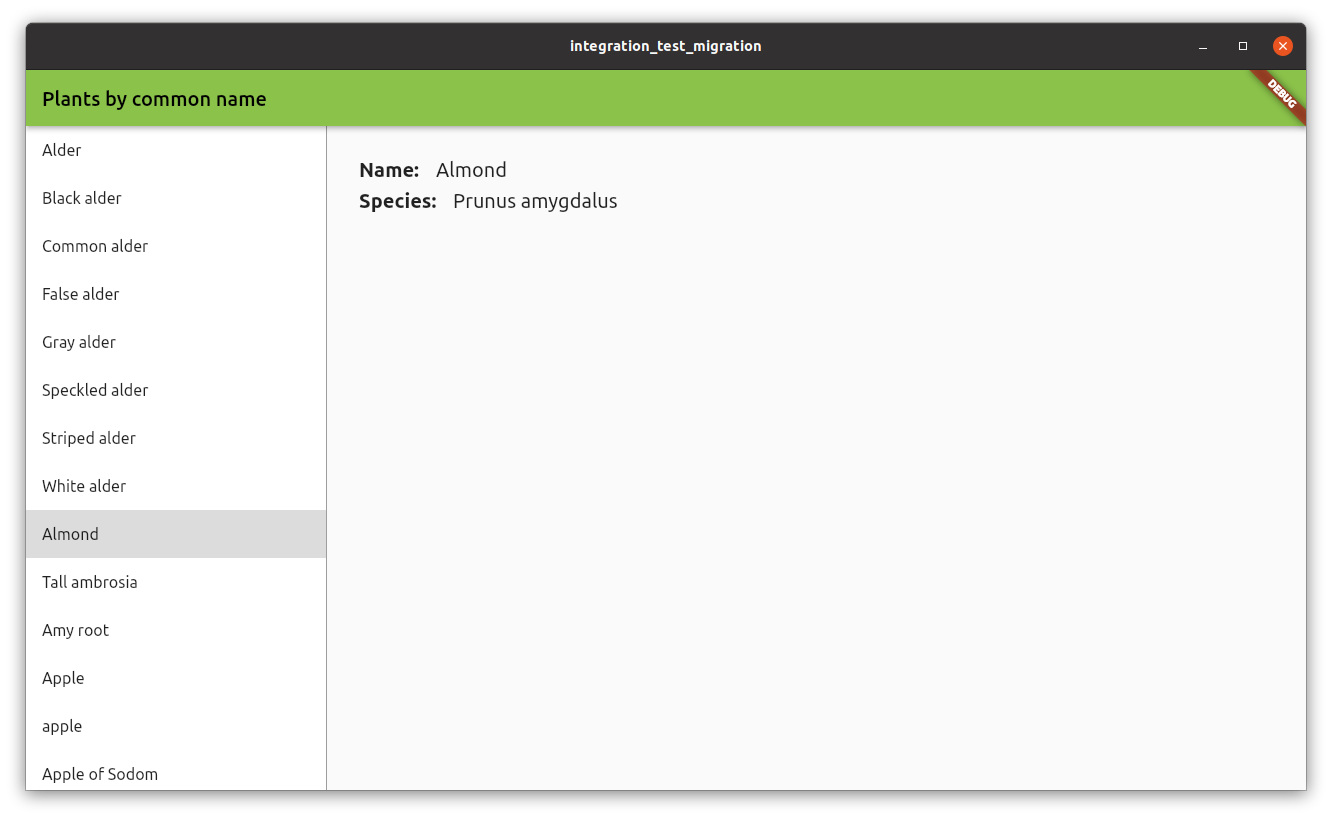
您可以在範例專案資料夾中找到完整的程式碼範例。
現有測試
#該專案包含三個 flutter_driver
測試,執行以下檢查:
- 驗證應用程式的初始狀態。
- 選擇植物列表中的第一個項目。
- 捲動並選擇植物列表中的最後一個項目。
這些測試包含在 test_driver
資料夾內的 main_test.dart
檔案中。
在此資料夾中還有一個名為 main.dart
的檔案,其中包含對 enableFlutterDriverExtension()
方法的呼叫。使用 integration_test
時,不再需要此檔案。
設定
#若要開始使用 integration_test
套件,請將 integration_test
新增至您的 pubspec.yaml
檔案(如果尚未新增)。
dev_dependencies:
integration_test:
sdk: flutter
接下來,在您的專案中,建立一個新的目錄 integration_test/
,並在那裡建立您的測試檔案,格式為:<名稱>_test.dart
。
測試遷移
#本節包含如何將現有的 flutter_driver
測試遷移到 integration_test
測試的不同範例。
範例:驗證小工具是否顯示
#當應用程式啟動時,右側的畫面會顯示一段文字,要求使用者從清單中選擇一個植物。
此測試驗證是否顯示該文字。
flutter_driver
在 flutter_driver
中,測試使用 waitFor
,它會等待直到 finder
可以找到小工具。如果找不到小工具,測試將失敗。
test('do not select any item, verify please select text is displayed',
() async {
// Wait for 'please select' text is displayed
await driver.waitFor(find.text('Please select a plant from the list.'));
});
integration_test
在 integration_test
中,您必須執行兩個步驟:
首先,使用
tester.pumpWidget
方法載入主應用程式小工具。然後,使用
expect
和比對器findsOneWidget
來驗證小工具是否顯示。
testWidgets('do not select any item, verify please select text is displayed',
(tester) async {
// load the PlantsApp widget
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// Find widget with 'please select'
final finder = find.text('Please select a plant from the list.');
// Check if widget is displayed
expect(finder, findsOneWidget);
});
範例:點擊動作
#此測試對清單中的第一個項目執行點擊動作,該項目是一個帶有文字「Alder」的 ListTile
。
點擊後,測試會等待詳細資訊出現。 在這種情況下,它會等待顯示帶有文字「Alnus」的小工具。
此外,測試會驗證是否不再顯示文字「Please select a plant from the list.」。
flutter_driver
在 flutter_driver
中,使用 driver.tap
方法來使用 finder 對小工具執行點擊操作。
要驗證小工具是否未顯示,請使用 waitForAbsent
方法。
test('tap on the first item (Alder), verify selected', () async {
// find the item by text
final item = find.text('Alder');
// Wait for the list item to appear.
await driver.waitFor(item);
// Emulate a tap on the tile item.
await driver.tap(item);
// Wait for species name to be displayed
await driver.waitFor(find.text('Alnus'));
// 'please select' text should not be displayed
await driver
.waitForAbsent(find.text('Please select a plant from the list.'));
});
integration_test
在 integration_test
中,使用 tester.tap
來執行點擊動作。
點擊動作後,您必須呼叫 tester.pumpAndSettle
來等待直到動作完成,並且所有 UI 變更都已發生。
要驗證小工具是否未顯示,請使用相同的 expect
函數和 findsNothing
比對器。
testWidgets('tap on the first item (Alder), verify selected', (tester) async {
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// find the item by text
final item = find.text('Alder');
// assert item is found
expect(item, findsOneWidget);
// Emulate a tap on the tile item.
await tester.tap(item);
await tester.pumpAndSettle();
// Species name should be displayed
expect(find.text('Alnus'), findsOneWidget);
// 'please select' text should not be displayed
expect(find.text('Please select a plant from the list.'), findsNothing);
});
範例:捲動
#此測試與先前的測試類似,但會向下捲動並點擊最後一個項目。
flutter_driver
若要使用 flutter_driver
向下捲動,請使用 driver.scroll
方法。
您必須提供執行捲動動作的小工具,以及捲動的持續時間。
您還必須提供捲動動作的總偏移量。
test('scroll, tap on the last item (Zedoary), verify selected', () async {
// find the list of plants, by Key
final listFinder = find.byValueKey('listOfPlants');
// Scroll to the last position of the list
// a -100,000 pixels is enough to reach the bottom of the list
await driver.scroll(
listFinder,
0,
-100000,
const Duration(milliseconds: 500),
);
// find the item by text
final item = find.text('Zedoary');
// Wait for the list item to appear.
await driver.waitFor(item);
// Emulate a tap on the tile item.
await driver.tap(item);
// Wait for species name to be displayed
await driver.waitFor(find.text('Curcuma zedoaria'));
// 'please select' text should not be displayed
await driver
.waitForAbsent(find.text('Please select a plant from the list.'));
});
integration_test
使用 integration_test
,可以使用 tester.scrollUntilVisible
方法。
您不需要提供要捲動的小工具,而是提供您正在尋找的項目。 在這種情況下,您正在搜尋文字為「Zedoary」的項目,它是清單中的最後一個項目。
該方法會搜尋任何 Scrollable
小工具,並使用給定的偏移量執行捲動動作。 該動作會重複執行,直到項目可見。
testWidgets('scroll, tap on the last item (Zedoary), verify selected',
(tester) async {
await tester.pumpWidget(const PlantsApp());
// wait for data to load
await tester.pumpAndSettle();
// find the item by text
final item = find.text('Zedoary');
// finds Scrollable widget and scrolls until item is visible
// a 100,000 pixels is enough to reach the bottom of the list
await tester.scrollUntilVisible(
item,
100000,
);
// assert item is found
expect(item, findsOneWidget);
// Emulate a tap on the tile item.
await tester.tap(item);
await tester.pumpAndSettle();
// Wait for species name to be displayed
expect(find.text('Curcuma zedoaria'), findsOneWidget);
// 'please select' text should not be displayed
expect(find.text('Please select a plant from the list.'), findsNothing);
});
除非另有說明,否則本網站上的文件反映了 Flutter 的最新穩定版本。 頁面最後更新於 2024-05-03。 檢視原始碼或回報問題。